Create a Vite app with user authentication using the Identity Platform on GCP
Create a Vite app with user authentication using the Identity Platform on GCP
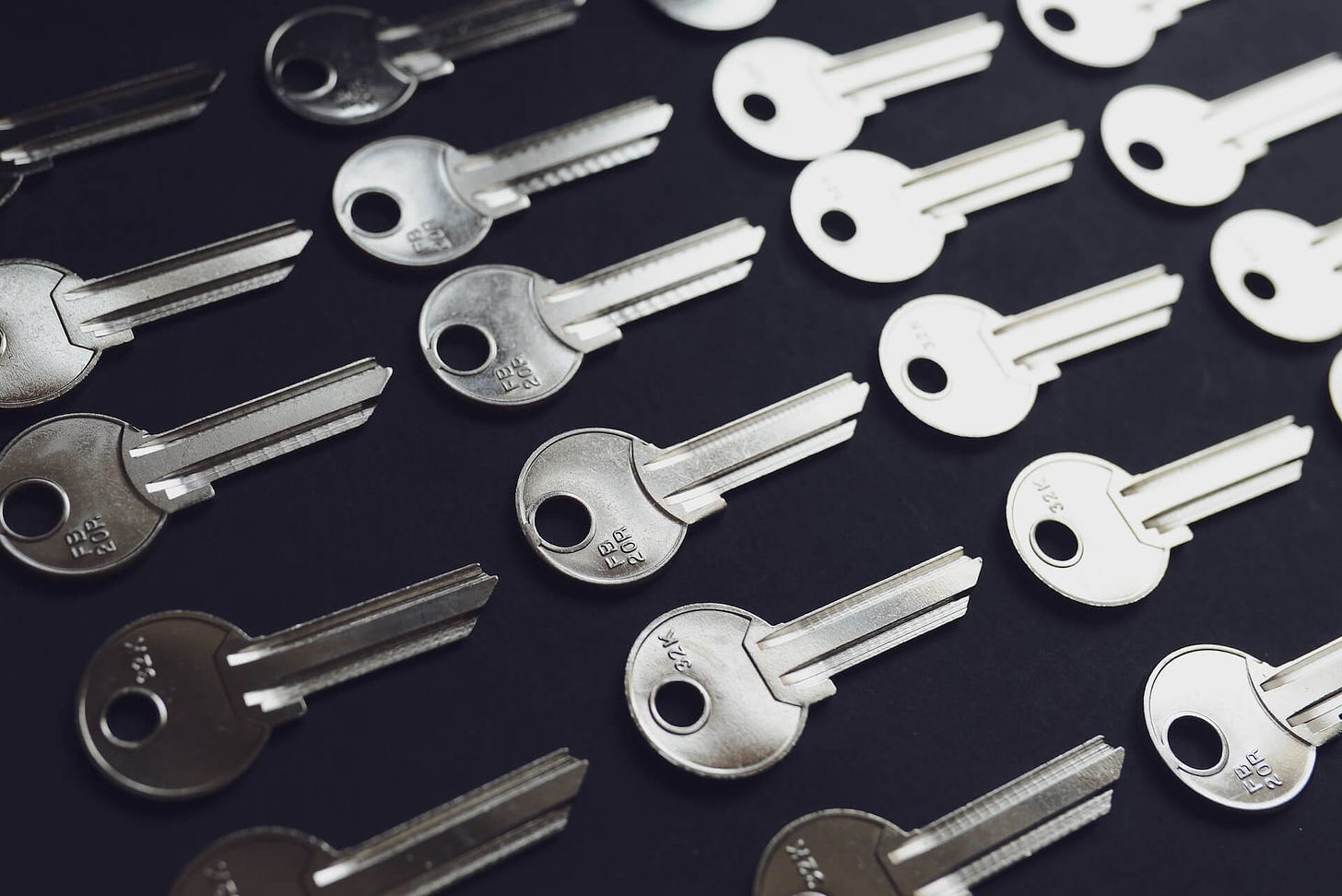
Introduction
Following on from my previous post on OAuth with Gin and React, I wanted to write another same same but different style post where instead of having to maintain any backend code, all the user authentication is handled completely serverlessly (in true serverless addict fashion) and all on the frontend. For example if you have say a blog, where you only want signed in users to be able to read certain posts or see some content where you want to protect it from public/anonymous users, then you could make use of this. Read on!
What is Vite and Identity Platform?
Vite, French for “fast”, (pronounced “veet”) is, true to it’s name, a lightweight and really fast front end framework for bootstrapping React applications. If you’re interested in learning more, I’d highly encourage you to check out Vite!
Identity Platform is an offering from Google Cloud which allows you to setup and manage users at any scale for your apps so you don’t need to manage it yourself. From the website:
Identity Platform is a customer identity and access management (CIAM) platform that helps organizations add identity and access management functionality to their applications, protect user accounts, and scale with confidence on Google Cloud.
Demo repository
Rather that walking you through boilerplate setup code and say copy this, then create that etc., I’ve create a repo from which you can either fork or use as a template to get going a lot faster. So instead I’ll walk you through the usage of the repo. Find the repository here.
How to use it
Let’s start off by looking at the App.tsx
file. Most of it is boilerplate you get when you create a new Vite app, however, looking just under the function declaration we can see the useAuthState
hook which comes with the Firebase SDK. Short note: We don’t actually need to use Firebase here, however the docs currently point to using the Firebase SDK to access a number of convenience methods and helpers. From the hook we access three variables.
user
loading
error
Then towards the bottom of App.tsx
, we’ve got some checks in place to validate the signed in user, and to display their name if signed in or if the hook is still loading, or if there’s an error. Simple react stuff, that if you’re a React developer should be pretty straight forward.
If you’re not signed in and there’s no errors or the app isn’t loading then we present an option to press a button to sign the user in. The button has a onClick
handler which calls signInWithGoogle
Let’s dive into the GoogleProvider.tsx
file which has this function.
In this 21 line file, we’ve got some placeholders for setting up an API Key and AuthDomain in an object called Firebase Config. When you enable the Google Cloud project to use Identity Platform, it will give you these values which you can populate here for your app.
We then export two things from this file, the auth object and the function to handle the Sign In With Google function, which the button in the App.tsx
file calls.
Getting the API keys and AuthDomain for the config.
In the console, once you’ve enabled the API, navigate to https://console.cloud.google.com/customer-identity/providers which will list out the available providers you’ve setup for your application. If you haven’t already got Google as a sign-in provider, follow the prompts to add it via clicking on the “Add Provider” button.
Once completed you’ll see a button called “Setup Details” under the “Configure your application” heading. Click setup details and you’ll get a popup which will have the apiKey
and authDomain
values for your app. If you get a bit lost, there’s a handy guide here.
Acting on the Sign In button
When the user presses the sign in button, a popup will display and then on completion of the sign in, a token will be stored in your browsers local storage which the react application can use to determine if you’re signed in or not, as well as get information about the signed in user such as the user’s name or email address.
As you can see in the App.tsx
on line 22, we welcome them by name with:
{user && <h2>Welcome {user.displayName}</h2>}
Conclusion
In this post we learned how to use Vite and Identity Platform to create a react application which allows the user to sign in to your application using Google Sign in. We talked about how the sign in button works and how the user information and whether or not a user is signed in or not can help us determine what to show to our end users. I hope you found this useful and welcome any comments or feedback. You can either get in touch with me directly via the contact page or on twitter. If you’d like to raise an issue or PR on the repo, I would love to hear about it!